Downloading SharePoint files might be necessary in areas, where we have lack of good network bandwith, if you want to keep working e.g. when you travel by train or plane. Downloading sharepoint files can be time consuming if you have to do it one by one. In this article, you will learn, how you can do it easily in the GUI, but also how you can download your SharePoint files with a so called SharePoint Downloader – a PowerShell script. If you are only interested in the SharePoint downloader, click here.
Table of Contents
Download SharePoint files in the GUI
Before we start doing this with PowerShell, I want to show you how you download the file in the GUI. GUI stands for graphical user interface. I will show you how to download the file Opportunity2 from the SharePoint Library Opportunitylibrary. This is absolutely sufficient in our daily work routine.
In the first step we open a SharePoint library:
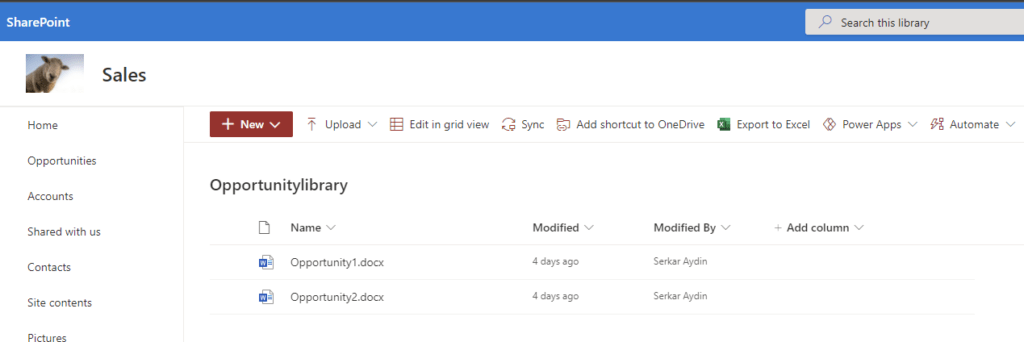
Here we can mark a file and then download – looks easy right?
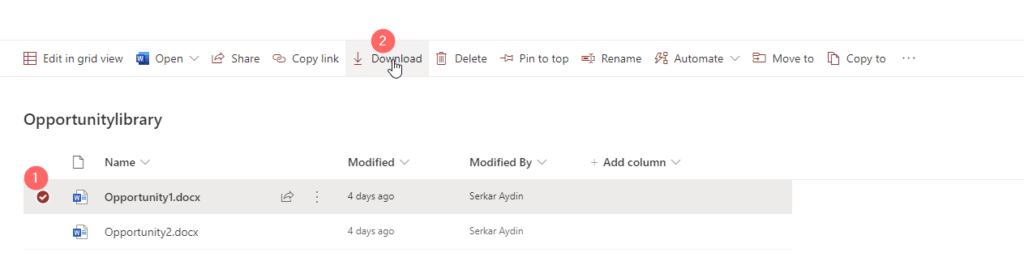
As you can see the file is downloaded and we can keep working offline – but hey don’t forget to upload it later to SharePoint again :).
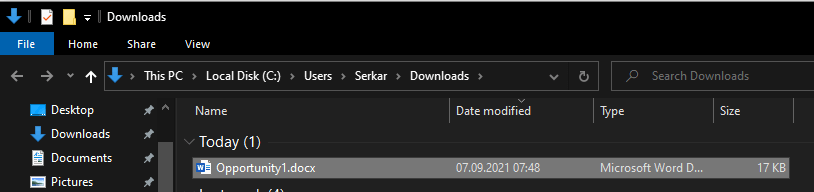
Download SharePoint files with PowerShell
Scenario
If you need to download multiple SharePoint files – this makes sense, if you need the files in another system and you need to export the files periodically, you can do it with PNP.PowerShell. In the following scenario, I will show you how to download multiple files by the example of the Library “Opportunitylibrary”, which contains two documents.
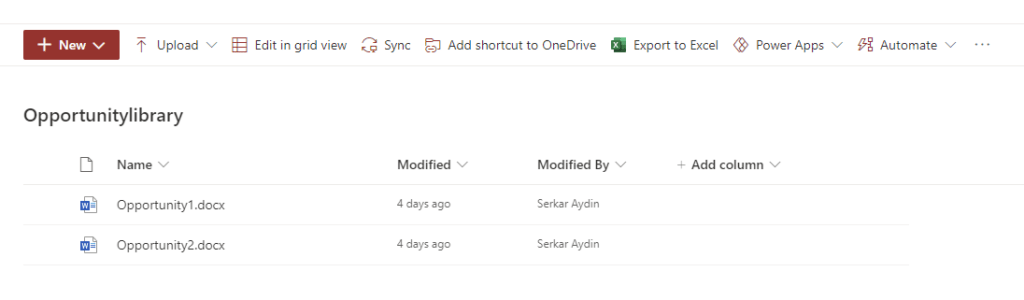
Authentication and parameters
In the beginning, we start with connecting to the site. If you are a beginner, you might be interested in a detailed description, which you can find here: Connect to SharePoint with PowerShell | SharePoint Online (sposcripts.com)
I have exported the credential object previously to reuse it, which you also can do. This is strongly recommended if the script shall ran in the background e.g. when you want to make use it in task scheduler. Do not expose the credentials in blank text – this is very bad practise and is a security problem. If you want to make use of saved credentials like I did, check the article: Use credentials in PowerShell | SPO Scripts
Before you run the scripts, change the parameters to your siteurl, your path, your list and your credential file. The parameters I have provided won’t work, if you don’t change them.
param ( $SiteUrl = "https://devmodernworkplace.sharepoint.com/sites/Sales", $DownloadPath = "C:\temp", $ListName = "Invoices", $CredPath = "C:\Users\Serkar\Desktop\devmod.key" ) $Credential = Import-Clixml -Path $CredPath Connect-PnPOnline -Url $SiteUrl -Credential $Credential
For interactive PowerShell sessions, you can also choose this authentication mechanism. Interactive means, that a person is running the script and can interact with the PowerShell terminal. The opposite would be if you would schedule the task in the task scheduler and the task would run – let’s say once a day. For this purpose the script would ask the terminal, but since there is nobody responsing the authentication request, the script would be halted and no file would be downloaded.
param ( $SiteUrl = "https://devmodernworkplace.sharepoint.com/sites/Sales", $DownloadPath = "C:\temp", $ListName = "Invoices" ) Connect-PnPOnline -Url $SiteUrl -Interactive
Download all SharePoint files from a library with PowerShell
If you want to download all files of a library, you have to run following script. The script iterates through all files in your SharePoint library defined in the parameter block and it will download all the files to the downloadpath you have provided in the parameter block.
Get-PnPListItem -List $ListName | ForEach-Object { Get-PnPFile -Url $_.FieldValues.FileRef -Filename $_.FieldValues.FileLeafRef -Path $DownloadPath -AsFile }
As you can see, I could download SharePoint files with PowerShell.
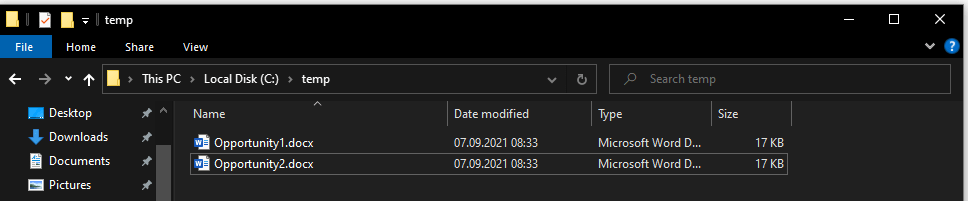
Download specific SharePoint files from a library with PowerShell
If you want to download only specific files, you can use following script. This script is using the out-gridview cmdlet to give you the possibility to choose only specific files.
Get-PnPListItem -List $ListName | Select-Object Id,@{N="FileName";E={$_.FieldValues.FileLeafRef}}, @{N="Link";E={$_.FieldValues.FileRef}} | Out-GridView -PassThru | ForEach-Object { Get-PnPFile -Url $_.Link -Filename $_.FileName -Path $DownloadPath -AsFile }
A grid will pop up, where you can select the documents, which you want to download. I have marked the second file and clicked on OK. If we check the folder, we can see, that the file have been downloaded!
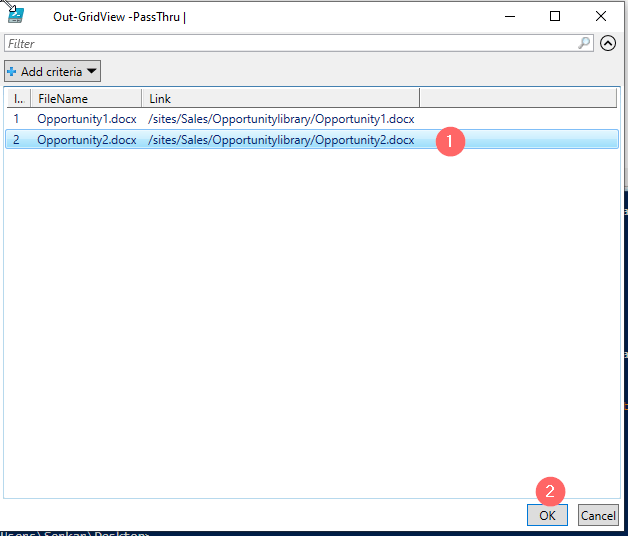
Et voilà – the file, which I have marked is downloaded!
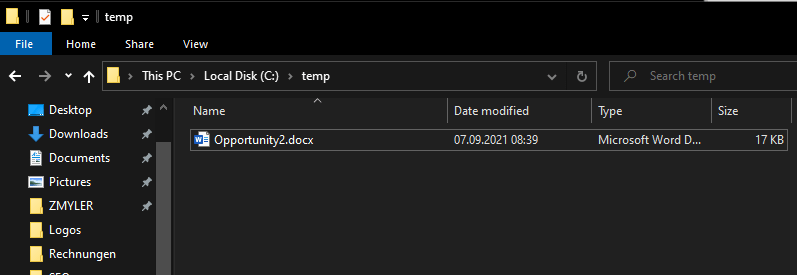
SharePoint Downloader
In this section I provide you my ready to run scripts. I call them SharePoint Downloader – a small tool with big impact 🙂
SharePoint Downloader for automation: Ready-to-run script for automation
With this script you can download SharePoint files to your specified folder. It’s purpose is for automation, means that it can run in the background with azure automation triggered by a runhook or with task scheduler triggered by a time schedule. Please change the parameters of the SharePoint Downloader and ensure, that the credential object is exported to the CredPath before scheduling the script. If you forgot how to do it, check out this article: Use credentials in PowerShell | SPO Scripts
param ( $SiteUrl = "https://devmodernworkplace.sharepoint.com/sites/Sales", $DownloadPath = "C:\temp", $ListName = "Opportunitylibrary", $CredPath = "C:\Users\Serkar\Desktop\devmod.key", $Force = $true ) $Credential = Import-Clixml -Path $CredPath Connect-PnPOnline -Url $SiteUrl -Credential $Credential #create folder if it does not exist If (!(Test-Path $DownloadPath)) { New-Item -ItemType Directory $DownloadPath } If ($Force) { Get-PnPListItem -List $ListName | ForEach-Object { Get-PnPFile -Url $_.FieldValues.FileRef -Filename $_.FieldValues.FileLeafRef -Path $DownloadPath -AsFile -Force } } Else { Get-PnPListItem -List $ListName | ForEach-Object { Get-PnPFile -Url $_.FieldValues.FileRef -Filename $_.FieldValues.FileLeafRef -Path $DownloadPath -AsFile } }
SharePoint Downloader for interactive: Ready-to-run script for interactive sessions
This script can be used for interactive sessions, so you need personally to start the script and not the task scheduler or azure automation. You will be asked for each parameter, when starting the script.
param ( [Parameter( Mandatory=$true, ValueFromPipelineByPropertyName=$true, Position=0, HelpMessage="Please provide the url in this format: https://tenant.sharepoint.com/sites/sitename") ] $SiteUrl, [Parameter(Mandatory=$true, ValueFromPipelineByPropertyName=$true, Position=1, HelpMessage="Please provide path, where the downloaded files should be located at.") ] $DownloadPath, [switch][Parameter(Mandatory=$false, ValueFromPipelineByPropertyName=$true, Position=2, HelpMessage="Use -Force paramater to overwrite existing files.") ] $Force = $true ) Connect-PnPOnline -Url $SiteUrl -Interactive #create folder if it does not exist If (!(Test-Path $DownloadPath)) { New-Item -ItemType Directory $DownloadPath } $ListName = Get-PnPList | Out-GridView -PassThru | Select-Object Title -ExpandProperty Title If ($Force) { Get-PnPListItem -List $ListName | Select-Object Id,@{N="FileName";E={$_.FieldValues.FileLeafRef}}, @{N="Link";E={$_.FieldValues.FileRef}} | Out-GridView -PassThru | ForEach-Object { Get-PnPFile -Url $_.Link -Filename $_.FileName -Path $DownloadPath -AsFile -Force -WarningAction Stop Write-Host "Downloaded $($_.FileName) Green to $DownloadPath" -ForegroundColor Green } } Else { Get-PnPListItem -List $ListName | Select-Object Id,@{N="FileName";E={$_.FieldValues.FileLeafRef}}, @{N="Link";E={$_.FieldValues.FileRef}} | Out-GridView -PassThru | ForEach-Object { Get-PnPFile -Url $_.Link -Filename $_.FileName -Path $DownloadPath -AsFile -WarningAction Stop Write-Host "Downloaded $($_.FileName) Green to $DownloadPath" -ForegroundColor Green } }
Conclusio
With PNP.PowerShell we can download SharePoint files easily. In this article I have described, how you can download single and multiple SharePoint files with the GUI and with PowerShell. I have provided you the SharePoint Downloader – a script, which downloads all files in SharePoint library interactively or automated.
References
Here is the official reference from microsoft about the main cmdlet get-pnpfile: Get-PnPFile (PnP.Powershell) | Microsoft Docs
Pingback: Sometimes you have to download SharePoint files for integration purposes – Learn how do it fully automated. – 365 admin service