In this article, I want to show you how to get SharePoint List Items with Graph API. Sometimes we are limited to the tools we can use, so we need to think about other approaches, to get our aim delivered. In this article, I want to show you how you can get SharePoint List items with the Microsoft Graph API by using PowerShell.
To get SharePoint List Items with Graph API, you can follow the next three steps:
- Configure the Azure Enterprise Application
- Grant the Enterprise Application the Permission
- Get SharePoint List Items with Graph API in PowerShell
Table of Contents
Prerequistes
- You need to install the PNP PowerShell module for step 2:
Connect to SharePoint with PowerShell | SharePoint Online (sposcripts.com) - You will need the consent of a global administrator for the enterprise application
Step 1: Configure the Azure Enterprise Application
I am following the least privilege approach and grant only the necessary permission for the app registration.
Hence I have created an App registration with following permissions:
Permission Name | Type |
Sites.Selected | Application |
User.Read | Delegated |
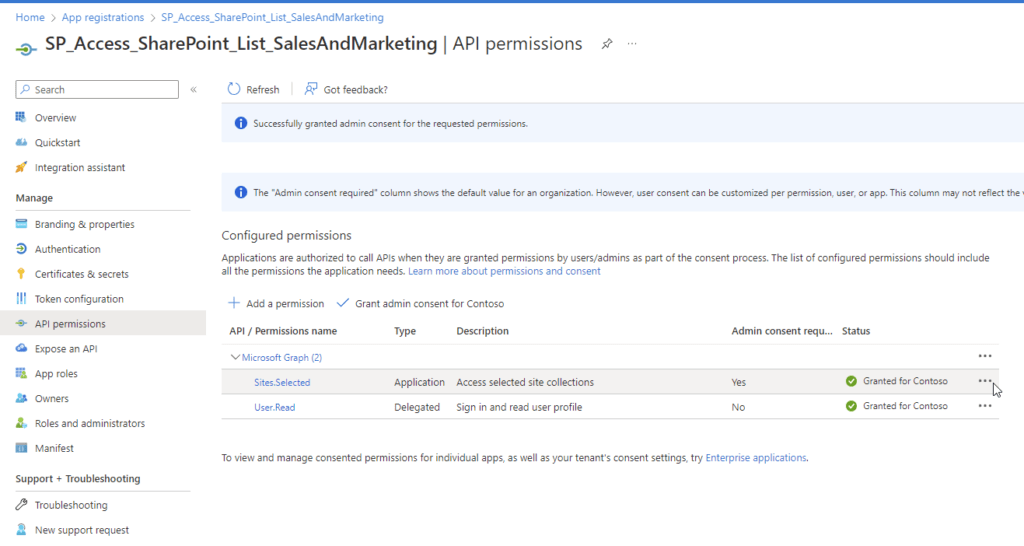
If you don’t know how to create it, follow my next steps, otherwise if you are familiar with it, you can also skip to the Step 2 – Grant the Enterprise Application the Permission.
1. Browse to Azure Portal and Search for Application Registrations and click on New registration
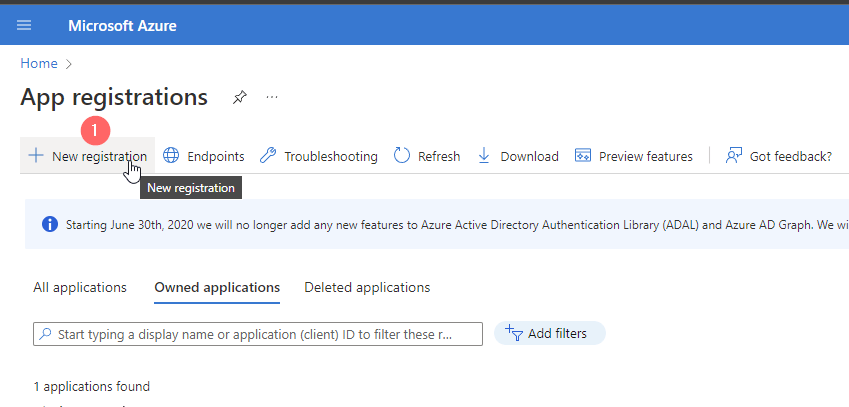
2. Give the App a meaningful name, which follows your organization standards (Differnt admins should recognize what the purpose for this app is) and Register it
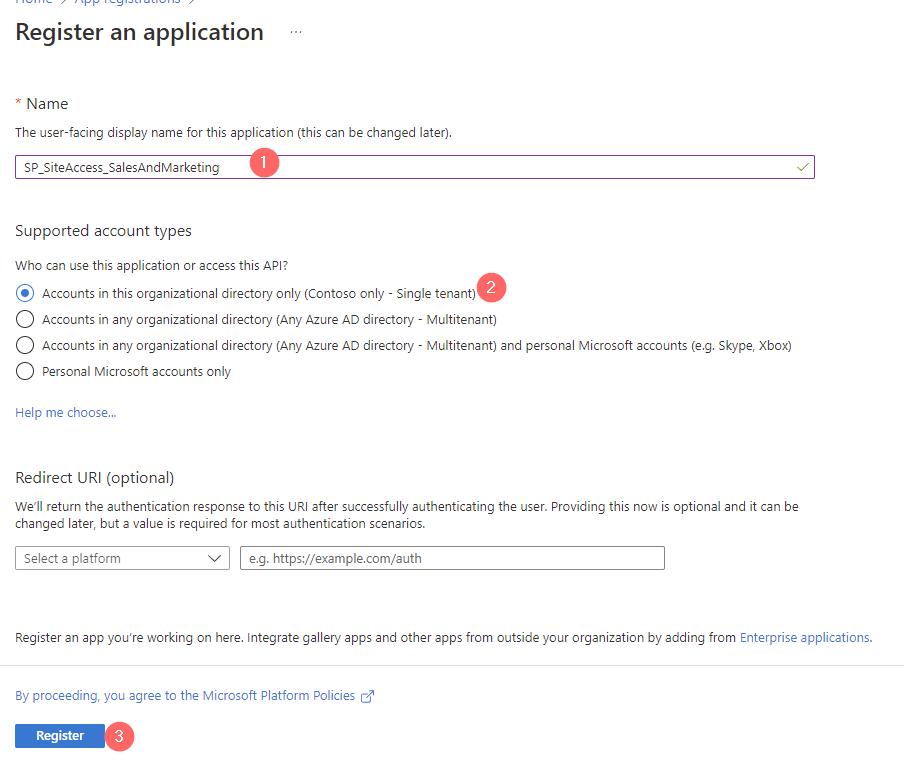
3. Note down the Application ID and go to Certificates & Secrets
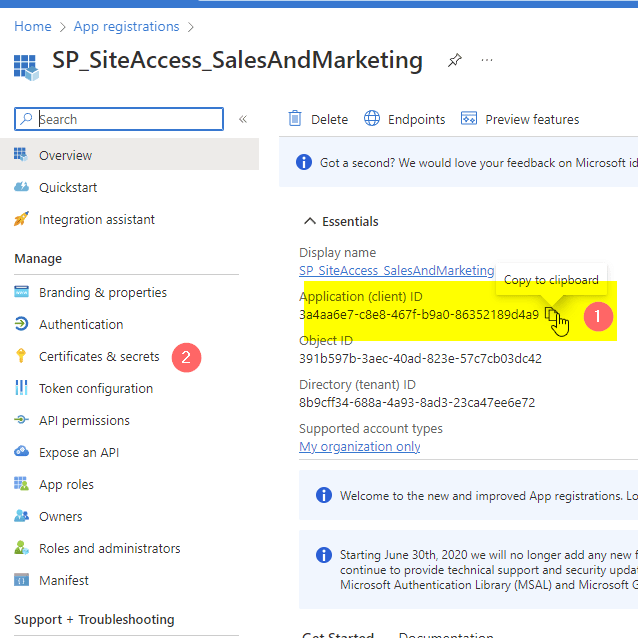
4. Create a new client secret or upload a certificate (I will show the secret approach)
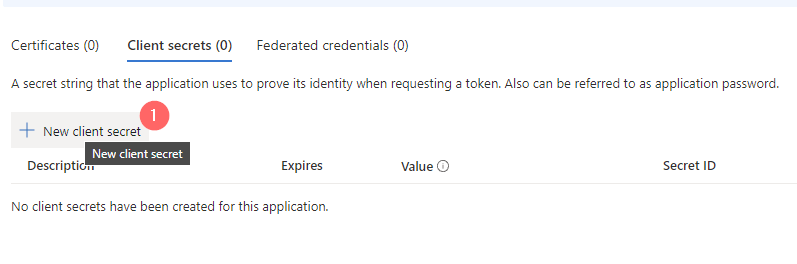
5. Also here a meaningful name is supportive for other colleauges. For the duration it makes sense to go with a reasonable duration. I would go with the recommendation of Microsoft as you might have lost this application out of sight in 24 months, which is the maximum duration for a client secret.
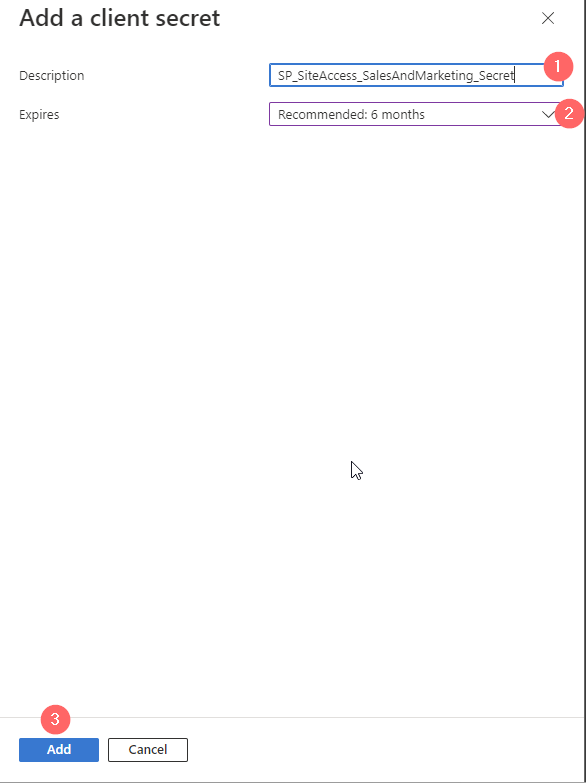
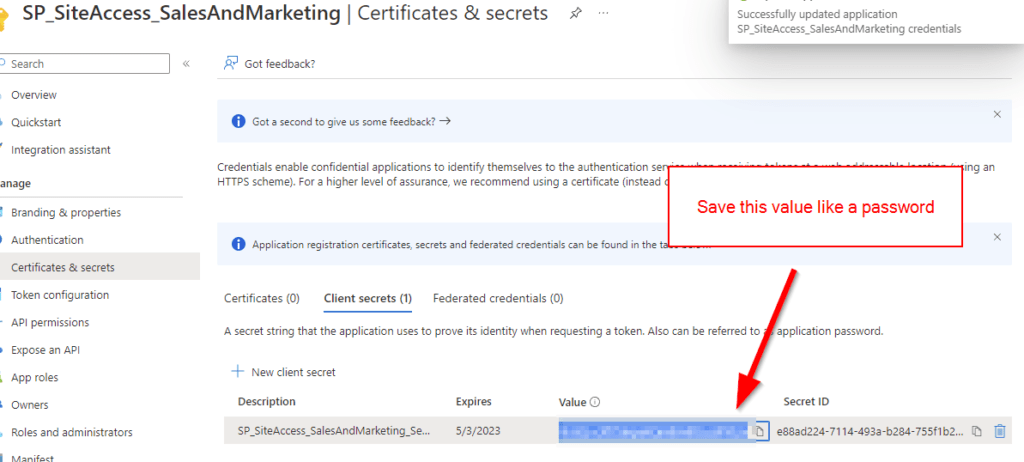
7. Now Click on API permissions on the left navigation pane and add a permission for Microsoft Graph
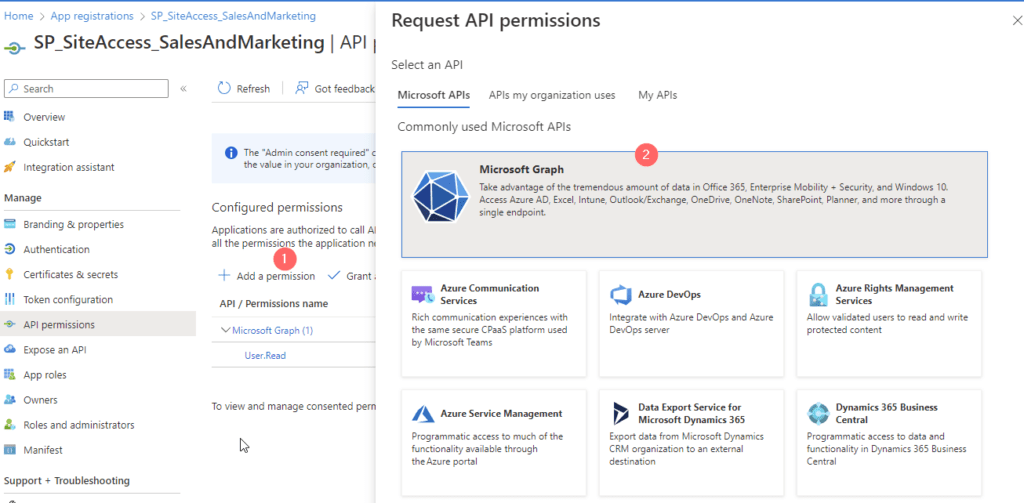
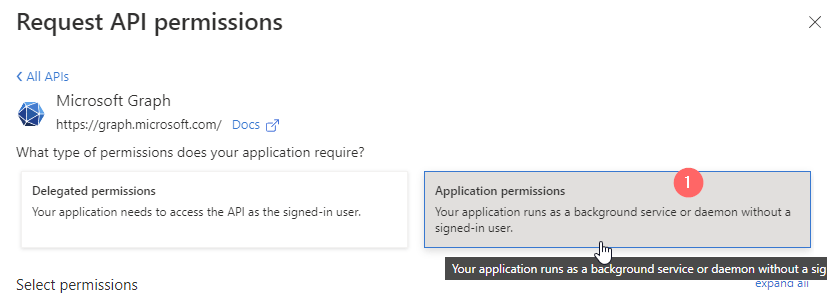
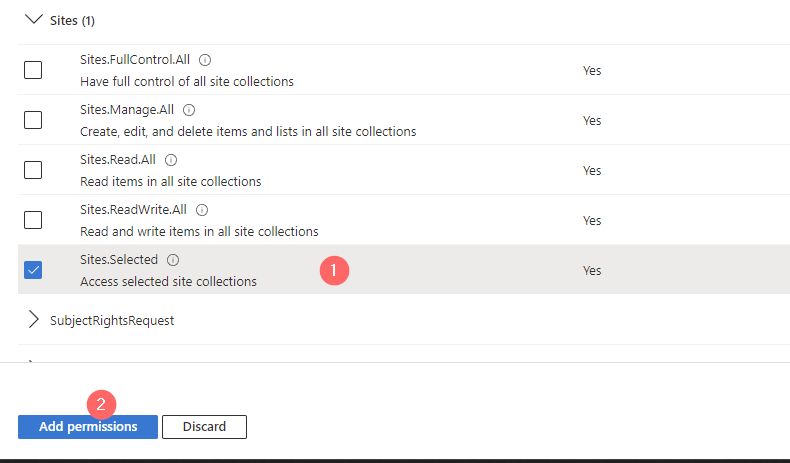
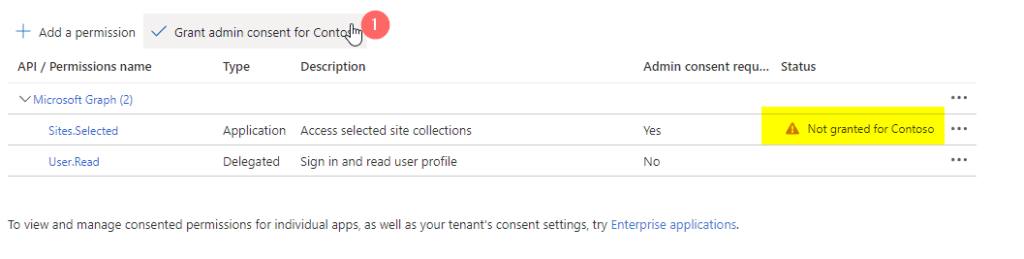
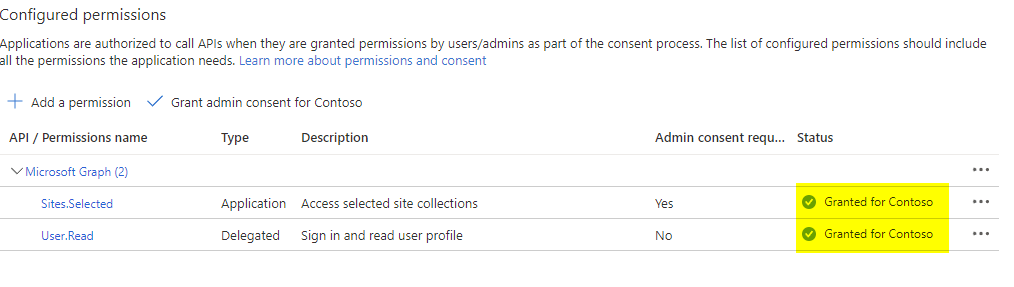
That’s it. You created an Azure App registration with Sites.Selected permission, where you need now to grant the permissions for the specific site.
Step 2: Grant the Enterprise Application the Permission
For this step you need to ensure that PNP Module is installed on your client and that you are allowed to use it.
If both conditions are appliying, you can use this code to grant Enterprise App, created in Step 1 the right permission for the site. In this case I am granting a read role.
Import-Module PnP.PowerShell $AppID = "9ea2120f-288c-47b6-8895-31e0fb4d9211" $DisplayNameofSitePermission = "Enterprise Application SP_Access_SharePoint_List_SalesAndMarketing" $SiteURL = "https://m365x323732.sharepoint.com/sites/SalesAndMarketing" Connect-PnPOnline -Url $SiteURL -Interactive Grant-PnPAzureADAppSitePermission -AppId $AppID -DisplayName $DisplayNameofSitePermission -Site $SiteURL -Permissions Read
You will need to log in with an account, which has access to the site.
After that you will see, that the Enterprise Application has now read access to the Site.
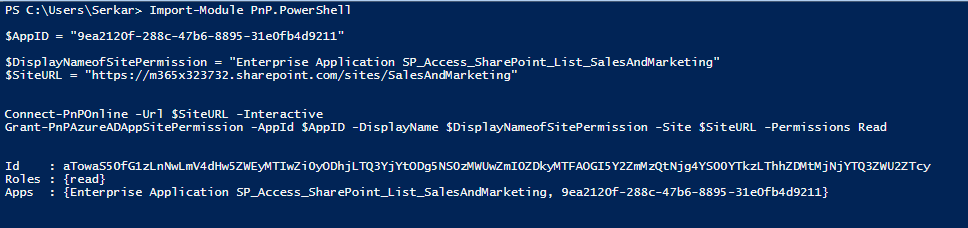
Step 3: Get SharePoint List Items with Graph API in PowerShell
As the enterprise application has now the permission to read contents from the designated SharePoint Site, you are able to read the contents of the SharePoint Site.
For this we need the app credentials and the site id of the site for which you want to read out the content.
<siteurl>/_api/site/id
For my example it is:
https://m365x323732.sharepoint.com/sites/SalesAndMarketing/_api/site/id

Once you adjusted the parameters, you can run the script
Param ( $AppID = "9ea2120f-288c-47b6-8895-31e0fb4d9211", $Scope = "https://graph.microsoft.com/.default", $Tenant = "m365x323732", $SiteID = "e35cee33-6d10-4e2c-a83b-496a26062ad3", $ListTitle = "Product%20List" ) Import-Module PnP.PowerShell $AppCredential = Get-Credential($AppID) #region authorize $Body = @{ client_id = $AppCredential.UserName client_secret = $AppCredential.GetNetworkCredential().password scope = $Scope grant_type = 'client_credentials' } $GraphUrl = "https://login.microsoftonline.com/$($Tenant).onmicrosoft.com/oauth2/v2.0/token" $AuthorizationRequest = Invoke-RestMethod -Uri $GraphUrl -Method "Post" -Body $Body $Access_token = $AuthorizationRequest.Access_token $Header = @{ Authorization = $AuthorizationRequest.access_token } #endregion #region get items $GraphUrl = "https://graph.microsoft.com/v1.0/sites/$SiteID/lists/$ListTitle/items?expand=fields" $Items = Invoke-RestMethod -Uri $GraphUrl -Method 'GET' -Body $Body -Headers $Header $Items.value.fields #endregion
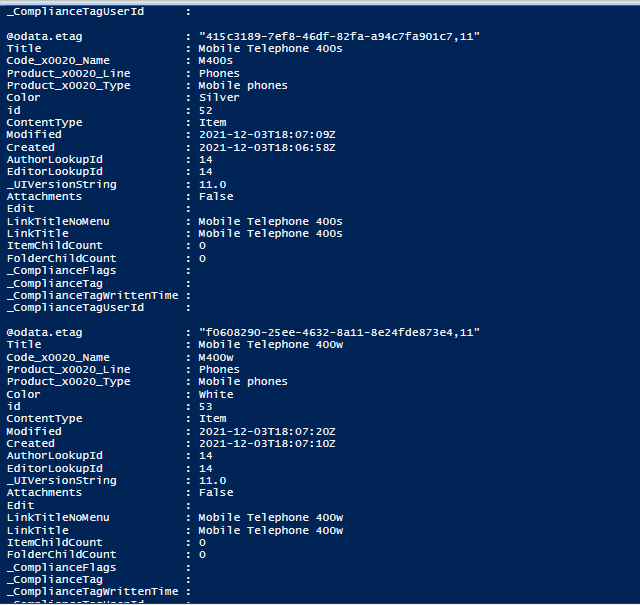
Further reference
To understand how Microsoft Graph API works, check out this article from Microsoft:
Use the Microsoft Graph API – Microsoft Graph | Microsoft Learn
You might also be interested in getting all SharePoint lists with PowerShell
SharePoint Online: How to get all lists and libraries (sposcripts.com)